API¶
The rogue_scroll
module¶
- class rogue_scroll.Constants¶
A class for constants used in this module.
- N_SCROLLS = 18¶
Type:
int
- N_SYLLABLES = 147¶
Type:
int
- SCROLL_KINDS = ('monster confusion', 'magic mapping', 'hold monster', 'sleep', 'enchant armor', 'identify potion', 'identify scroll', 'identify weapon', 'identify armor', 'identify ring, wand or staff', 'scare monster', 'food detection', 'teleportation', 'enchant weapon', 'create monster', 'remove curse', 'aggravate monsters', 'protect armor')¶
Type:
tuple
[str
, …]
- SCROLL_PROBS = {'aggravate monsters': 3, 'create monster': 4, 'enchant armor': 7, 'enchant weapon': 8, 'food detection': 2, 'hold monster': 2, 'identify armor': 7, 'identify potion': 10, 'identify ring, wand or staff': 10, 'identify scroll': 10, 'identify weapon': 6, 'magic mapping': 4, 'monster confusion': 7, 'protect armor': 2, 'remove curse': 7, 'scare monster': 3, 'sleep': 3, 'teleportation': 5}¶
Type:
dict
[str
,int
]
- SYLLABLES = ['a', 'ab', 'ag', 'aks', 'ala', 'an', 'app', 'arg', 'arze', 'ash', 'bek', 'bie', 'bit', 'bjor', 'blu', 'bot', 'bu', 'byt', 'comp', 'con', 'cos', 'cre', 'dalf', 'dan', 'den', 'do', 'e', 'eep', 'el', 'eng', 'er', 'ere', 'erk', 'esh', 'evs', 'fa', 'fid', 'fri', 'fu', 'gan', 'gar', 'glen', 'gop', 'gre', 'ha', 'hyd', 'i', 'ing', 'ip', 'ish', 'it', 'ite', 'iv', 'jo', 'kho', 'kli', 'klis', 'la', 'lech', 'mar', 'me', 'mi', 'mic', 'mik', 'mon', 'mung', 'mur', 'nej', 'nelg', 'nep', 'ner', 'nes', 'nes', 'nih', 'nin', 'o', 'od', 'ood', 'org', 'orn', 'ox', 'oxy', 'pay', 'ple', 'plu', 'po', 'pot', 'prok', 're', 'rea', 'rhov', 'ri', 'ro', 'rog', 'rok', 'rol', 'sa', 'san', 'sat', 'sef', 'seh', 'shu', 'ski', 'sna', 'sne', 'snik', 'sno', 'so', 'sol', 'sri', 'sta', 'sun', 'ta', 'tab', 'tem', 'ther', 'ti', 'tox', 'trol', 'tue', 'turs', 'u', 'ulk', 'um', 'un', 'uni', 'ur', 'val', 'viv', 'vly', 'vom', 'wah', 'wed', 'werg', 'wex', 'whon', 'wun', 'xo', 'y', 'yot', 'yu', 'zant', 'zeb', 'zim', 'zok', 'zon', 'zum']¶
Type:
list
[str
]
- class rogue_scroll.Generator(min_syllables: int = 1, max_syllables: int = 3, min_words: int = 2, max_words: int = 4, separator: str = '') None ¶
Rogue scroll information.
- Parameters:
min_syllables (
int
, default:1
)max_syllables (
int
, default:3
)min_words (
int
, default:2
)max_words (
int
, default:4
)separator (
str
, default:''
)
- DEFAULT_MAX_S = 3¶
Type:
int
- DEFAULT_MAX_W = 4¶
Type:
int
- DEFAULT_MIN_S = 1¶
Type:
int
- DEFAULT_MIN_W = 2¶
Type:
int
- DEFAULT_SEPARATOR = ''¶
Type:
str
- static count_possibilities(n: int, min: int, max: int) int ¶
\(\displaystyle\sum_{x=\mathrm{min}}^{\mathrm{max}} n^x\)
Note that this can return 0. Keep that in mind if you wish to take the logarithm of the result.
- Raises:
ValueError – if min > max.
ValueError – if n < 1.
- Parameters:
n (
int
)min (
int
)max (
int
)
- Return type:
int
- entropy() float ¶
Entropy in bits.
- Raises:
ValueError – if no titles would be possible
- Return type:
float
- random(with_entropy: bool = False) Scroll ¶
Generate a random Scroll.
If
with_entropy
is True, the entropy, computed at generation time, will be included in theScroll
.- Parameters:
with_entropy (
bool
, default:False
)- Return type:
- classmethod random_kind() str ¶
Randomly picks a scroll kind using weighted probabilities.
- Return type:
str
- random_title() str ¶
Generate random scroll title.
- Return type:
str
- class rogue_scroll.Scroll(title: str, kind_index: int, entropy: float | None = None) None ¶
A scroll as a title and a kind
- Parameters:
title (
str
)kind_index (
int
)entropy (
Optional
[float
], default:None
)
- property entropy¶
The entropy from how the scroll was generated if known
If the entropy wasn’t computed when the scroll was generated then this returns ‘None’
- property kind¶
Returns what kind of scroll it is.
- property title¶
Returns the title of the scroll
Warning: Amgiguous titles and entropy¶
Reported entropy will be higher than the true value under default settings.
This is because in some cases there may be multiple ways to generate the same title word. Consider the two syllable word “abit
”. That could have been generated as either “a
” + “bit
” or as “ab
” + “it
”.
Use --syllable-divider
(-d
) with the rogue-scroll
command or specify the separator
parameter when calling Generator
to something that is not a letter or a space.
Examples¶
Note that because output is random, it is a bit tricky to contrive doctests.
from rogue_scroll import Generator, Scroll, Constants
Entropy computations are not random are a function of the parameters used to create a Generator
g = Generator() # default values
H = g.entropy()
print(f"{H:.2f}")
86.44
An example in which we generate between 4 and 6 words, inclusive
g = Generator(min_words = 4, max_words = 6)
for _ in range(5):
title = g.random_title()
word_count = len(title.split())
assert word_count >= 4 and word_count <= 6
print(f'"{title}" has {word_count} words')
That might produce an output such as
"ulk rhovmonbie uni orgrhov con ha" has 6 words
"potfucomp zeburta zok neriteklis" has 4 words
"bjorapp alaha bek biebekso fuuniu" has 5 words
"alapo ninan hyditsne ple" has 4 words
"erkbublu rhov alala arzeshunelg" has 4 words
We also create scrolls of various kinds using the probability weights from the orginal game.
for _ in range(5):
k = Generator.random_kind()
assert k in Constants.SCROLL_KINDS
print(f'a scroll of {k}')
That would produce an output that looks something like,
a scroll of hold monster
a scroll of identify ring, wand or staff
a scroll of identify ring, wand or staff
a scroll of scare monster
a scroll of enchant armor
One could check that scroll kinds are picked with a probability corresponding
to SCROLL_PROBS
by building on something like,
hist = {s: 0 for s in Constants.SCROLL_PROBS.keys()}
trials = 1000
for _ in range(trials):
s = Generator.random_kind()
hist[s] = hist[s] + 1
# we will just look for a few
row_format = "{:<20} {:>5} {:>10}"
print(row_format.format("Kind", "Count", "Expected"))
for k in ['protect armor', 'monster confusion', 'identify potion']:
expected = round(Constants.SCROLL_PROBS[k] * trials / 100)
count = hist[k]
print(row_format.format(k, count, expected))
That would yield results with values something like this,
Kind Count Expected
protect armor 19 20
monster confusion 69 70
identify potion 95 100
When that example is fleshed out with more useful reporting and run with 10000 trials we get a result like
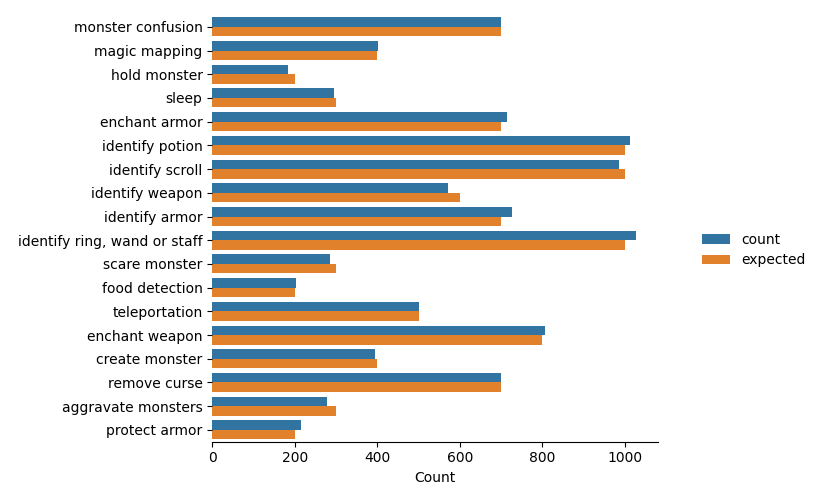
Reusts from one run with picking 10,000 scroll kinds. Kolmorogov-Smirnov test statistic is 0.17.¶